The first thing we have to do is add a Google map to our web page, if you are unfamiliar with this step please refer back to my post How to add a basic Google map API to your website. Your basic Google maps code without a marker should look similar to this.
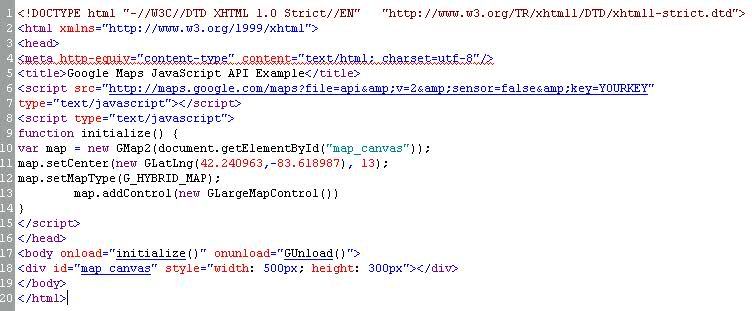
The next step in the process is to add the point to your map with a marker. Here is the code to do that.
var point = new GLatLng(42.251012,-83.625011);
var marker = new GMarker(point);
map.addOverlay(marker);
GEvent.addListener(marker, "click", function() { alert ("Hello World"); });
Code Broken Down
var point = new GLatLng(42.251012,-83.625011);
This line of code creates a variable called "point", and sets the exact point where you want to place the marker on your map
var marker = new GMarker(point);
Here you are creating a variable called "marker", and then preparing google maps to use the coordinates of your "point" in this marker
map.addOverlay(marker);
This adds the actual marker to the map
GEvent.addListener(marker, "click", function() { alert ("Hello World"); });
As an added bonus, you can add an onclick event handler to the marker and call a function that displays an alert
You can find the final product here, don't foget to click the marker :)
This is a very basic way of adding a point to a Google map, there are many ways to utilize markers into your website. Yelp uses markers to mark business locations, this website uses markers to dynamically show the coordinates of your selected point of the map. I look forward to seeing your creativity at work.
Enjoy!!
Josh, That is a great explanation (as always)of how to add markers to google maps. Although, I do like the search function which automatically places markers on the map based on what you search for. I may have to keep your Blog posts for reference when I develop my own website.
ReplyDeleteTerry,
ReplyDeleteThis is a very basic example of adding a marker, it gets trickier when you start cycle through coordinates and add several markers at once.
Josh,
ReplyDeleteGreat post.. I added Google maps to my web page, but haven't done anything with it. It is functional I just don't have any markers pointing to locations or search bar as of yet. I still haven't decided what to do since Drake changed the requirements for iteration 3. Your blog will be helpful if I decide to do more with Google maps.
Josh, thanks for that excellent post. Sharing knowledge eases all our life for this class and in the real-life.
ReplyDeleteWe thought of avoiding using google-maps for our group project. But maybe I'll put Google Maps into my individual one...
Josh as usual this is an excellent post. All of your post are mini tutorials that one has to save for later use.
ReplyDeleteI love how nice Google makes everything. We followed a similar process (in combination with Yelp) in order to plot our points on the map. You did a good job breaking down the code here.
ReplyDeleteThis is helpful. I have used that but still working on adding info window to display information from an xml file! Great post
ReplyDeleteI am with Dane, thanks to you and other students posting these great blogs, without them I do not think I would be able to do what I have completed.
ReplyDelete